PYTHON FULL STACK DEVELOPER (WEB)
Module 1: Basics of Web Development – Duration: 2 weeks
Week 1: Introduction to Web Development
- Understand how the internet works, HTTP/HTTPS, URLs.
- Learn about the client-server architecture.
- HTML: Study the structure and basic tags.
- What is HTML?
- Structure of HTML
- Types of Webpages
- HTML Tags
- Types of tags
- Explanation about html, head and body tags
- Input controls and attributes
- How to divide web page with div tag
- Formatting Tags
- Link Tags
- How to create order list and unordered list
- Table tag
- Form tag
- Color tag
- Frame Tag
- Div Tag
- Span
- Attributes
CSS: Learn about styling and layout.
- What is CSS?
- Advantages of CSS
- Why CSS?
- Syntax and Anatomy of CSS
- Types of Stylesheets
- External style sheet
- Internal style sheet
- Inline style
- Types of Selectors
- Class
- Id
- Group
- Element
- Universal
- Styling
- What is style object?
- Properties of style
- Applying styles to elements
Week 2: Advanced HTML and CSS
Study HTML5 and its new elements.
- HTMLS5 Introduction
- HTML5 HISTORY
- New Features and groups
- Backward Compatibility
- Why HTML5?
- Power of HTML5:
- Common Terms in HTML5
- HTML5 Syntax
- The DOCTYPE:
- Character Encoding
- HTML5 New Elements
- HTMLS5 Video
- Introduction
- Video on the Web
- How It Works?
- Video Formats and Browser Support
- HTML5 Video Tags
- HTMLS5 Audio
- Introduction
- Audio on the Web
- How It Works?
- Audio Formats and Browser Support
- HTML5 Audio Tags
- HTMLS Input Types
- Introduction
- color
- date
- Div’s & Span tags
- Background
- background-color
- background-image
- background-repeat
- background-attachment
- background-position
- Font
- font-family
- color
- font-size
- font-style
- fontvariant
- font-weight
- Text
- letter-spacing
- Word-spacing
- line-height
- text-decoration
- text-transform
- Dive deeper into CSS: selectors, specificity, box model, flexbox, and grid.
Module 2: JavaScript & Bootstrap– Duration 2 Weeks
Week 3-4:
- Introduction of javascript
- Cross browser issues.
- Declaration syntax of javascript
- Statements
- Comments
- Popup Boxes
- Alert
- Confirm
- Prompt
- Variables, Arrays and Operators
- Variables
- Operators
- Arithmetic
- Assignment
- Comparison
- Logical
- Document object model
- Functions and types
- Conversion functions
- Conditional statements
- if
- if…else
- if…elseif…else
- Switch
- Loops
- while
- do…while
- for
- for…in Statement
- Break
- Continue
- Window object Document object Arrays
- Associative Arrays
- Array Properties and Methods
- Advanced JavaScript
- Date object
- this object
- Event object
- State management
- Cookie
- Form validation
- Expressions
- Email validation
- Dynamic functionalities of html controls
- Project
Module 3: Back-End Development (Python, Django/Flask/Rest API & Database)
(SQLite/MySQL) – Duration: 4 Weeks
- Week 5-6: Python – Core
- Introduction to Python
- Installation of Python on Windows, Linux
- Setting Python Environment
- Using Python Interpreter (Interactive Mode lDLE)
- Python fundamentals (Multiline Statements and Comments)
- Variables and Basic Data Types
- Python Variables
- Numbers and Boolean
- Strings
Python Operators
- Arithmetic Operators
- Relational Operators
- Assignment Operators
- Logical Operators
- Membership Operators
- Identity Operators
- Bitwise Operators
- Conditional Statements
- Indentation and Related Errors
Loops in Python
- for Loop
- while Loop
- Nested Loop
- Usage of Continue, Break and Pass keywords
More on Data Types and In-Built Functions
- String and its functions
- List and its functions
- Tuple and its functions
- Set and Frozen Set and its functions
- Dictionary and its functions
- Comprehensions (List, Dictionary)
Functions in Python
- Introduction to function (Defining and calling functions)
- Styles of writing functions
- Types of return statements
- Types of arguments (Required, Default, Keyword, Variable Length (Tuple and Dictionary)
- Variable Scope – Global vs Local
- Types of Functions (recursive and anonymous functions)
- More on Lambda Function – map(), reduce(), zip() filter()
Modules in Python
- Understanding modules
- Importing standard modules
- Usage of in-built modules (String, Math, Datetime, Random, Sys, Os, functools)
- Locating modules
- Creating module
- File Operation in Python
- What is a file?
- Modes in file (read, write, append, binary modes)
- Methods in file operations
- File Handling using OS (creating & changing directory, rename & deleting file)
- Command Line inputs (using sys)
Module 4: Python – Advanced
Week 7-8:
- OOPs in Python
- Introduction to OOPs
- Classes & Objects
- Methods and attributes (user defined methods, constructor, meaning of self, access modifiers)
- Static variables and methods
- Inheritance and its types
- Method Overriding
- Operator overloading
- Exception Handling
- What are Errors and Exceptions?
- Types of in-built Exceptions
- Handling an Exception (try, except, finally, else)
- Raising an Exception
- Custom Exception
- Regular Expressions
- Usage of Regular Expressions (using re)
- Decorators
- Iterators & Generators
- Working with Json file
PDBC
- Multi threading & Multi processing
- Pyodbc (Python ODBC Module)
- Install Pyodbc Module
- Getting The Database Connection Details
- Connecting To SQL Server Database
Pandas
- Introduction to Pandas and Environment setup
- Introduction to Pandas Data structures
- What is a Series, DataFrame, Panel?
- Creating Pandas DataFrames
- Preview and examine data in a Pandas DataFrame
- Selecting and Manipulating Data
- Exporting and Saving Pandas Data Frames
- Additional useful functions
NumPy
- Introduction to NumPy and Environment setup
- NumPy NDARRAY object
- NumPy Data types
- NumPy Array Attributes, Array Creations
- NumPy Indexing and Slicing
- NumPy arithmetic and statical functions
- NumPy- Sort, Search and Counting functions
- NumPy — matrix library
Module 4: Django & Database(Sqlite/MySQL) – Duration 4 Weeks
Week 9-12:
- What is Django?
- Why Django? Key Advantages
- History of Django
- Features of Django
- Characteristics of Django
- Companies Using Django
- Difference b/w MVC and MVT
- Models Views and Templates
- Web Frameworks
- What is a Web Framework?
- What is a server?
- HTTP Requests and HTTP Responses
- What is a web framework ?
- Django Architecture
- Django Installation
- Virtual Environment
- Working with Pycharm
- Working with ATOM
- Developing First Django Application
- Django Project Architecture
- Exploring manage.py,
- Exploring urls.py
- Exploring settings.py,
- Exploring admin.py,
- Exploring models.py,
- Exploring views.py,
- Application creations and Examples
- Django Application Creation
- Steps in Application creation
- Working with views
- Working with HTML and CSS
- Working with Bootstrap
- Django Application creation in Atom
- Django Application creation in Pycharm
- project with multiple Applications
- Reusing a Application in different projects
- Working with Static files
- Django Views
- Requesting a web page via URL
- Rendering web page via view function
- Render Http Response to templates
- Application with multiple views
- Understanding context object and dictionary type
- GET and POST methods
Django Templates
- Template tags
- Template Filters
- Template API
- Passing Dynamic content to template file
- Passing multiple dict values to template
- Loading static files
- Adding image file to template
- Advanced Templates
- Template library
- custom template filter
- custom templates tags
- Registering the tags
- Django Admin
- Activating the Admin interface
- Creating super user for Admin site
- Using the Admin site
- How to use the Admin site
Django Models
- Working with models and databases
- Defining Models
- Model Fields
- Defining forms
- Model Forms
- Make migrations and migrate
- Registering models in settings.py
- Registering models with Admin site
- Advanced Concepts
- Django ORM
- Faker Module
- Class based views
- Form validation
- Rendering forms
- crispy forms
- Multiselect Field
- Embedded Video
- Uploading and downloading Files
- Working with Audio and video
- Integrating with legacy databases and applications
- Using the Admin site
- How to use the Admin site
- Sessions users Registrations
- Security
- Django Deployment
- Other Contributed Frameworks
Introduction to SQL
- Introduction to Databases and RDMBS
- Install a Database Engine
- SQL Syntax
- SQL Data Types
- SQL Operators
- SQL Expressions
- SQL Comments
- SQL – Data Definition Language Commands
- DDL Operations
- SQL – Data Manipulation Language Commands
- DML Operations
- SQL – Data Control Language Commands
- DCL Operations
- SQL Functions
- SQL Queries and Sub Queries
- SQL Clauses
- SQL Joins
- SQL Views
- SQL Indexes
- SQL Transactions
- SQL Injection
- Project
Instructor
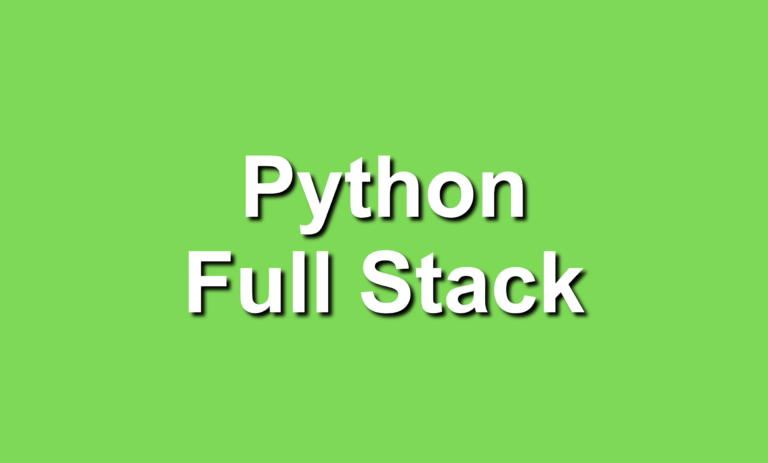