JAVA COURSE CONTENT
1. CORE JAVA
1.1. Introduction to Java
1.2. Java History
1.3. Why Java
1.4. Features of Java
1.5. Environment Setup
1.6. Hello World Application
1.7. Java Program Internal
1.8. JDK, JRE, JVM
1.9. JVM- Java Virtual Machine
1.10. Class, Objects, Data Types
1.11. Variables
1.12. Type Casting
1.13. Operators
1.14. Associatively of operators
1.15 Java Comments
1.16. Java Coding Standards
1.17. Package – Creating package, naming package
1.18. Java Keywords
1.19. Access Specifiers – Public, Private, Protected, Default
1.20. Static Concept – Block, Variable, Method, Class
1.21. Scanner Class
1.22. Control Statement – If, If…else, Nested if…else and SwitchCase
1.23. Java Break Statement
1.24. Java Continue Statement
1.25. Flow Statement- for, while, do..while
1.26. Constructor- Default, Parameterized
1.27. Inheritance
1.28. Extends, super and this keyword
1.29. Method Overloading, Method Overriding
1.30. Interface
1.31. Abstract Class
1.32. Abstract Class vs Interface
1.33. Garbage Collection
1.34. Marker Interface
1.35. Serialization
1.36. Transient
1.37. Java instanceof
1.38. Instance initializer block
1.39. Volatile Keyword in Java
1.40. Exception Handling
1.41. Exception Handling- try, catch, throw, throws, finally
1.42. String Concept
String Basics
String Methods
1.43. String Buffer
1.44. String Builder
1.45. Custom Immutable Class
1.46. Java – Arrays
1.47. Collection Framework
– Collection Basics
– Iterator Interface
– Methods of Collection Interface
1. List
A] Array List B] Linked List C] Vector
2. Set
A] Hash Set B] Tree Set 3. Map
A] Hash Map B] Tree Map C] Hashtable
1.48. Collection Framework Advance Concepts
1. Comparable Interface
2. Comparator Interface
3. Collections class
4. Differentiate Comparable and Comparator
1.49. Java Enum
1.50. Date Concept
1.51. File Handling Concept
1. File Handling Basics
2. Create Folder
3. Create File- .txt. .pdf, .xlsx, .docs, etc
4. Write File
5. Read File
6. Delete File
1.52. Thread
1. Concept, Lifecycle
2. Extends Thread
3. Implement Runnable Interface
4. Thread Priorities
5. Thread Methods
1.53. Multithreading
1.54. Thread Synchronization
1. Synchronization
2. Object Locking
3. Inter Thread Communication
1.55. JDK 1.8.0 New Features with Hands-on
1. Lambda Expression
2. Functional Interface
3. Default Method in Interface
4. Static Method in Interface
5. Method References
6. Date Time API
7. Stream API
8. Collectors
9. For each Loop
10. String Joiner Class
11. Parallel Sort
12. Optional Class
Advanced Concepts
2. J2EE
2.1 JDBC
1. JDBC Introduction
2. JDBC Architecture
3. Database Overview
4. JDBC Basics
5. MySQL
6. Create Database
7. Create Table
8. Insert, Update, Delete
9. Truncate
10. SQL Join
• Inner Join
• Left Outer Join
• Right Outer Join
• Full Outer Join
11. Aggregate Function
1. MIN
2. MAX
3. AVG
4. SUM
5. COUNT
12. SQL HAVINGClause
13. GROUP BY
14. ORDER BY
15. SQLAliases
16. SQL LIKEOperator
17. SQL IN
18. SQL NOT IN
19. SQLBetween
20. SQL Null Values
21. SQL TOP
22. SQL LIMIT
23. SQL Stored Procedures
24. Java Application Using JDBC Connectivity
25. Handling SQLExceptions
26. DriverManager
27. ResultSet
28. Connection
29. Statement
30. Prepared Statement
31. Collable Statement
32. DB ConnectivitySteps
33. Store Image in SQL
34. Read Image in SQL
35. SQL Queries using SQLYog
36. JDBC CRUD Application
2.2 Servlet
2.2.1 Servlet Basics
2.2.2 Need of Server Side Programming
2.2.3 Servlet Life
Cycle 1 Init()
2 Service()
2.1 doGet()
2.2 doPost()
3 Destroy()
2.2.4 Servlet Hello World Application
2.2.5 Web.xml Structure
2.2.6 Servlet Directives- include(), forword(), sendRedirictive()
2.2.7 HttpServletRequest, HttpServletResponse in Servlet
2.2.8 Servlet and JDBC Integration
2.2.9 Servlet, HTML 5, MySQL-JDBC, Apache Tomcat using Real Time Login Application
2.2.10 Servlet, JSP, MySQL- JDBC, Apache Tomcat using Custom CRUDApplication
2.2.11 Servlet, JSP, MySQL- JDBC, Apache Tomcat using Custom Sign Up | Sign InApplication
2.1 JSP
2.1.1 JSP Basics
2.1.2 Creating dynamic Web content with JSP
2.1.3 Scriplet
2.1.4 Declaration
2.1.5 Servlet, JSP, MySQL- JDBC, Apache Tomcat Login Application
2.1.6 Servlet, JSP, MySQL- JDBC, Apache Tomcat using User Sign Up | Sign In Application
2.1.7 Servlet, JSP, MySQL- JDBC, Apache Tomcat Using Advanced Custom Project Implementation
Instructor
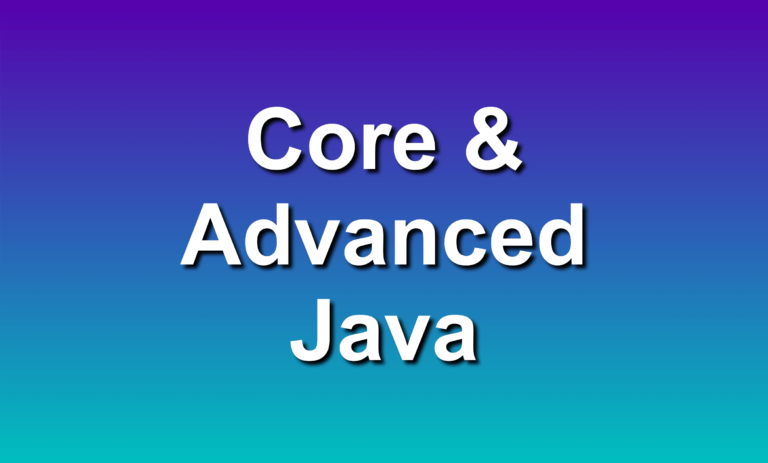